Recently I had the chance to incorporate the DFPlayer Mini into a project. It’s a super cute MP3 player that is great for Arduino powered projects. The only problem being with the documentation. It is a little spotty and I had to work pretty hard to get around a few issues. So here is a little cheat sheet to help you along:
How should I wire up the DFPlayer Mini to my Arduino?
If you dig into the pin map for the DFPlayer mini, you will find two different ways of driving a speaker. One set labeled ‘DAC left’ and ‘DAC right’ for use with an an inbuilt digital to audio converter. The other set labeled ‘SPK1 and SPK2’, and from the documentation looked like it is only useful for mono.
In practice, the SPK set supports stereo, and I found the DAC set to introduce a considerable amount of line noise. (Despite using the recommended 1K resistors between the Arduino and DFPlayer). Switching to the SPK set of outputs made a huge improvement in sound quality. I wired my DFPlayer mini up like this:
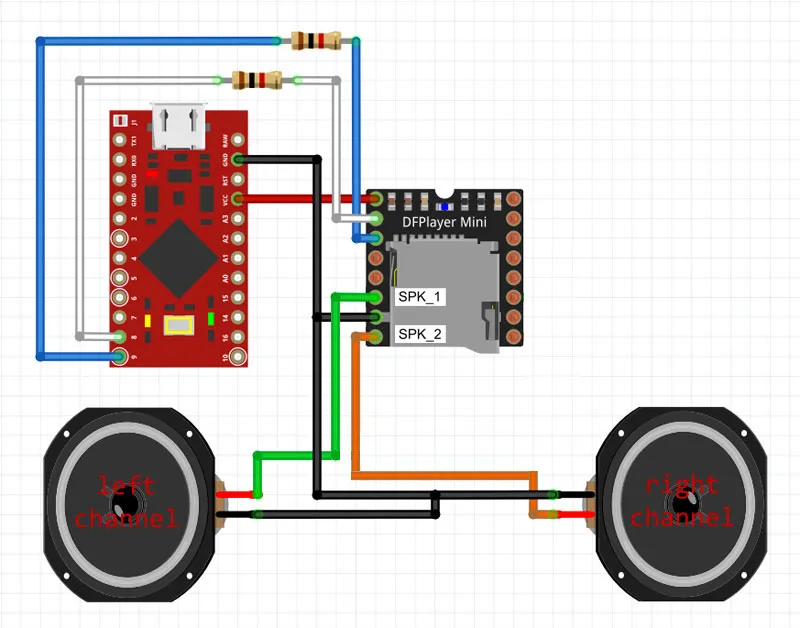
Oh and the polarity of a 3.5mm headphone jack? The pointy bit is the left channel, the middle the right and the part closest to the cable goes to ground.
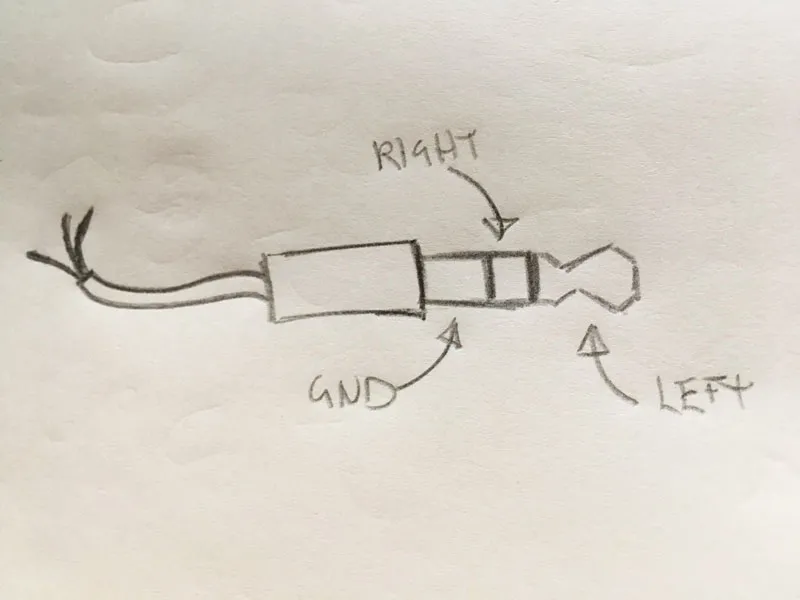
What library should I use with the DFPlayer Mini?
The DFRobotDFPlayerMini library for the most part works pretty well. It works great with software serial, which is what DFRobot use in their example code on their wiki.
The DFPlayer library has made my main loop very slow?
I found making a call to setVolume each time in the main loop to be fairly destructive on the loop performance. This call could take upwards of 100ms and, this made the frame-rate of some LED animation stuff that I was doing unacceptable.. In the end, I only called setVolume when the value of a potentiometer was adjusted. Playback volume is something that is only occasionally changed, and having a drop in animation performance during those times wasn’t noticeable.
uint8_t nVol = map(analogRead(volPin), 0, 1024, 0, 30);
if (nVol != state.vol) {
state.vol = nVol;
MP3Player.volume(state.vol);
}
How do I format and organise my micro SD Card to be compatible with the DFPlayer Mini?
Plug your micro SD card into your computer and then In OSX open up a terminal and use the following commands:
$sudo diskutil list
This will let you work out what logical disk is being used for your micro SD Card. In my case, I used the listed SIZE to work out that it was /dev/disk2. Now the card can be correctly formatted:
$sudo diskutil eraseDisk FAT32 MUSIC MBRFormat /dev/disk2
Now copy your MP3 files over to the micro SD card. They can go into a folder named ‘mp3’, but will work fine straight on the disk themselves. They must be sequentially numbered like so:
0001.mp3
0002.mp3
0003.mp3
…
You will also need to copy these files across in order, the DFPlayer seems to use some sort of creation timestamp when the files are index. So don’t copy 0003.mp3 and then 0001.mp3, otherwise wacky things will happen.
Finally, if you have done this all on OSX, you will need to clear out any hidden files as that will also mess up the DFPlayer’s indexing system.
$dot_clean /Volumes/Music
(Your volume folder might be different, depending on what you used as the disk name during the disk erase step).
I had a bunch of MP3 files and micro SD cards to produce, so I made a little script to make it a bit easier (I had all my sequentially numbered files on the desktop in a folder titled ‘soundFiles’):
#!/bin/bash
mkdir /Volumes/MUSIC/mp3
for filename in ~/Desktop/soundFiles/*.mp3; do
cp $filename /Volumes/MUSIC/mp3/
done
dot_clean /Volumes/MUSIC
DFPlayer Mini randomly locks up and doesn’t play
This one was really tricky to nail down, it only happened maybe 4% of the time I called ‘void play(int fileNumber)’? Everything was setup correctly the selected MP3 fie would play most of the time, but every now and then it wouldn’t. Super annoying. I had to write my own play method to work around the issue:
void playTrack(uint8_t track) {
MP3Player.stop();
delay(200);
MP3Player.play(track);
delay(200);
int file = MP3Player.readCurrentFileNumber();
Serial.print("Track:");Serial.println(track);
Serial.print("File:");Serial.println(file);
while (file != track) {
MP3Player.play(track);
delay(200);
file = MP3Player.readCurrentFileNumber();
}
}
This method makes sure any existing tracks are stopped first. Then we make sure that the current file number that the DFPlayer has matches the one we supplied. Keep trying till these track numbers match. It was the only way that I could play a track 100% of the time on the DFPlayer mini.
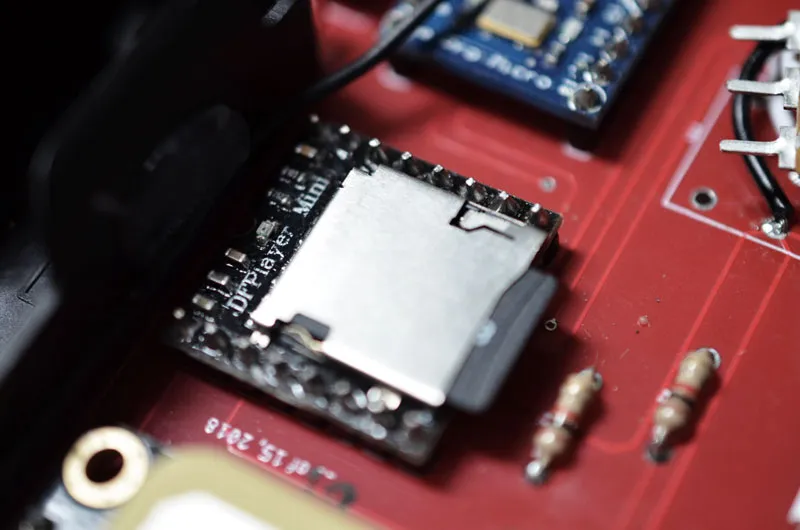
Edits:
- 2019-01-19: Updated schematic so that the speakers are wired to SPK1 and SPK2
Comments:
You can join the conversation on Twitter or Instagram
Become a Patreon to get early and behind-the-scenes access along with email notifications for each new post.
Hi! Subconsciously you already know this, but let's make it obvious. Hopefully this article was helpful. You might also find yourself following a link to Amazon to learn more about parts or equipment. If you end up placing an order, I make a couple of dollarydoos. We aren't talking a rapper lifestyle of supercars and yachts, but it does help pay for the stuff you see here. So to everyone that supports this place - thank you.